CSC111 Homework 9
This homework assignment is due on 4/8/10, at 11:59 p.m. plus 1 minute. It deals with while loops. |
Problem #1
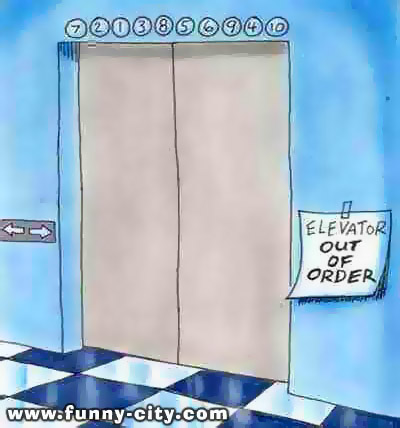
def main():
people = [ ("JT", 150), ("TJ", 80), ("TT", 120), ("BT", 170), ("Mike", 12), \
("AF", 27), ("Lea", 78), ("Leo", 180), ("Al", 55), ("GK", 110), \
("JR", 111), ("VB", 76) ]
Above is a list of people and their weight. They are waiting in front of an elevator. The maximum weight allowed on the elevator is 300 lbs.
Write a program called hw9a.py that will compute and output the answers to the following questions:
- Question 1
- What is the maximum number of people that can fit in the elevator?
- Question 2
- What is the total weight of the people who fit in the elevator?
- Question 3
- What is the name of the people in the elevator?
- Use while loops!
- Once your program works, modify it and make it input the total weight allowed in the elevator from the user. The allowed range allowed by your program should be any number between 0 and 1000. If the number entered by the user is less than 0 or greater than 1000, your program should prompt the user to input that number again.
- Make sure your program works if the maximum weight allowed is 0, or when it is set to 1000.
Recommendations
- A full 1.5 point will be given to documentation. Make sure you look at past solutions of homework assignments to see what is expected for the program header, and for in-line comments.
- Use functions!
Submission
- Submit your program as follows:
submit hw9 hw9a.py
Problem 2. Computing e
The mathematical constant e is equal to 2.718281828459045...
It can be computed by adding the terms of the infinite series: e = 1 + 1/1! + 1/2! + 1/3! + 1/4! + ... where 4! is the factorial of 4, and is equal to 4 * 3 * 2 * 1.
- How many terms do we need to sum up to get the first 6 digits of e correct, i.e. to obtain 2.71828?
- To answer this question, write a program that will compute and print out the answer. Store your program in a file called hw9b.py, and submit it as follows:
submit hw9 hw9b.py
- Pay attention to style and documentation, please!