CSC111 Homework 12 2015
--D. Thiebaut (talk) 17:30, 19 April 2015 (EDT)
Problem #1
Write a program called hw12_1.py that contains a recursive function called minmax() which returns the smallest and largest elements of a list of items. The items may be strings, numbers, or tuples, where the first element of the tuple is either a number or a string.
Example main() program:
def main(): A = [ 1, 10, 20, 3, 2, -1, -10, 5, 5, 5, 5 ] low, high = minmax( A ) print( "smallest item =", low, " largest item = ", high ) # will print # smallest item = -10 largest item = 20 A = [ 1 ] low, high = minmax( A ) print( "smallest item =", low, " largest item = ", high ) # will print # smallest item = 1 largest item = 1 A = [ ] low, high = minmax( A ) print( "smallest item =", low, " largest item = ", high ) # will print # smallest item = None largest item = None A = [ "alpha", "beta", "gamma", "epsilon" ] low, high = minmax( A ) print( "smallest item =", low, " largest item = ", high ) # will print # smallest item = alpha largest item = gamma if __name__=="__main__": main()
Requirements
- The function minmax() must be recursive.
- You cannot use the min() or max() standard Python functions. If you want to find the largest of two items, you need to do the comparison in Python, using <, <=, >, or >=.
Submission
Submit your program to the Moodle section, HW12 PB 1
Problem #2
Write a Python program called hw12_2.py that will generate a tree as close to the tree shown below as possible. You should not reproduce the text in the image; just the tree, its colors, and its fruit.
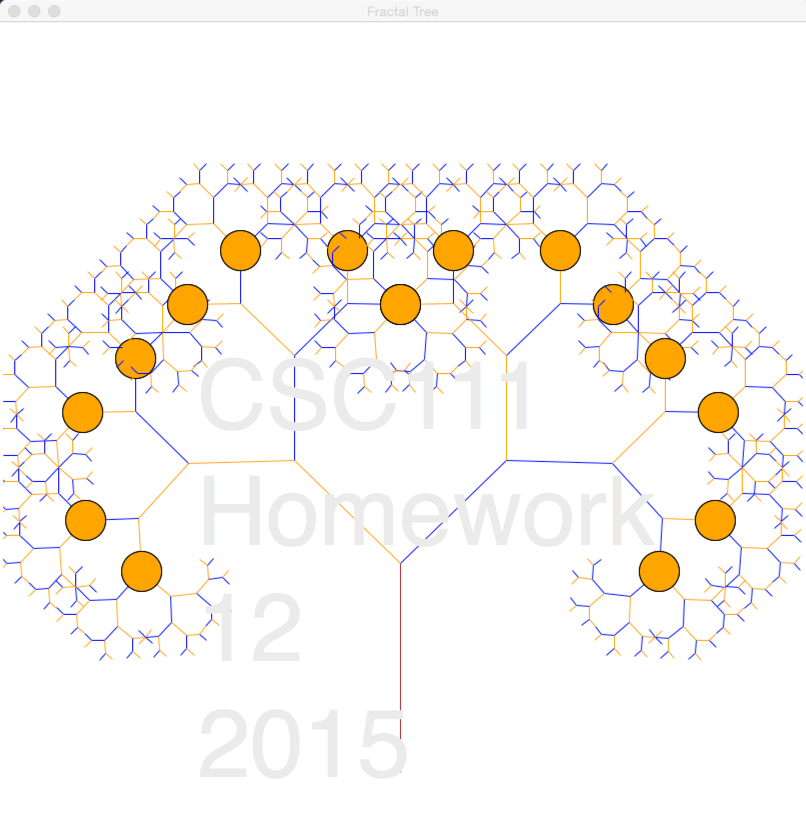
- Submit your program as well as a screen capture of its output to Moodle, in the HW12 PB2 and HW12 Image 2 sections.
- Note: To change the color of a line with the graphics library (use graphics111.py, please), you should use the setOutline() method rather than the setFill() method.
- I used only 3 colors to generate the tree above: "orange", "blue", and "brown".