CSC103 Assembly Language Lab 2014
--D. Thiebaut (talk) 21:48, 24 August 2014 (EDT)
This lab deals with assembly language and the Simple Computer Simulator (SCS). Work on this lab with a partner. It's easier to figure out some of these questions with another person. This lab uses numbers instead of variables when referring to memory.
Contents
The Simple Computer Simulator (SCS)
As you progress through the lab you will need some more information about the various instructions our SCS supports. These instructions are documented in this page.
Start
- Point your browser to the simulator to start it.
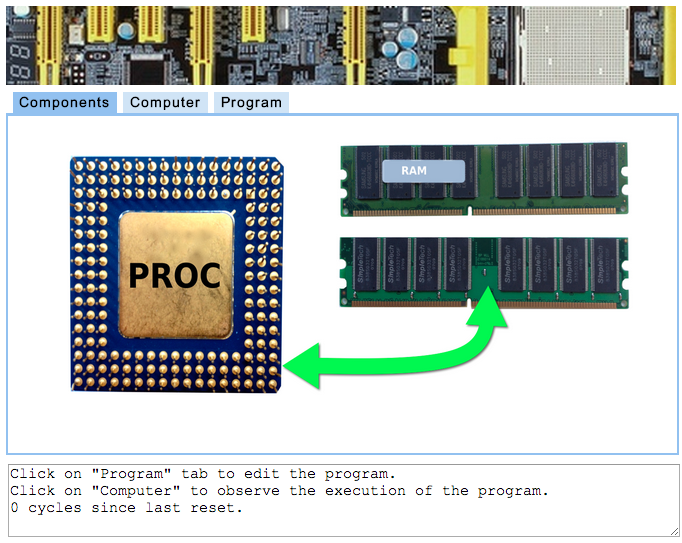
Writing the Program
- Click on the Program tab at the top of the simulator widget to open up the edit window.
- Highlight the default program that appears in the window and delete it.
- In the newly erased edit-window, enter this simple program:
LOAD 1
STORE [8]
HALT
You will notice that as you press the ENTER key after each line, the instruction is reformatted and a semicolon appended at the end. This is the normal of an instruction in assembly language. The number on the left is the next logical address where the instruction will be stored. The semicolon is a special marker that allows you to write a comment to give additional information about what you are trying to do. For example, we could have typed:
LOAD 1 ; load 1 into the accumulator
STORE [8]; store the accumulator at Address 8
HALT ; stop the program
After you press the ENTER key after the last line, you should get this listing in the edit-window (with or without comments, depending on whether you added them to your code):
0000: LOAD 1 ;
0002: STORE [8] ;
0004: HALT ;
Your program has been partly assembled. This is the term used to describe the translation that takes place when a collection of instructions gets transformed into numbers that will go into the memory of the computer. Here the numbers on the left of the colon represent the address in memory where each instruction will be saved.
Loading the Program into RAM
- Click on the Computer tab.
- Observe that all the instructions are there, written in purple, but that the actual contents of the memory are numbers. Each instruction is coded as a number, and each number is stored at an address in memory. The first instruction is always stored at Address 0. The first instruction of the program is LOAD 1, and LOAD is coded, as 4, stored at Address 0, and 1 is coded as... just 1, stored at the next address, i.e. Address 1. The STORE instruction is stored at Address 2, and its argument, 8, at Address 3. The final instruction, HALT is stored at Address 4.
Execute the Progam
Single Stepping
- Click on the button that says "Reset" to make sure the processor will execute the program starting at 0
- Click on the Step button and observe that the number 1 is going into the AC accumulator register and then into memory, at Address 8.
Running the program
- If you want to run the program full speed rather that executing it a step at a time, the procedure is slightly different.
- Click on Reset
- Change the contents of the AC register by entering some random number in the AC box, say 33.
- Click Run instead of Cycle. This runs the program.
- Observe that 33 is overwritten by 1, and then stored at Address 8.
- You now know the basics of programming in assembly language.
Problem 1
- Using the steps you have just gone through, write a program that initializes 3 memory locations at 10, 11, and 12 with the value 22. You should end up with the number 23 in three different memory locations.
If you get a message of the form:
- ***address already in use ***0002: STORE [9] ;
it means that you are attempting to put the instruction STORE [9] at Address 2, but this memory location is already used by another instruction. Simply remove the 0002: part of your line and you will be fine.
Problem 2
- This problem is very similar to the previous problem, but instead of storing the same value in all three variables, store the numbers 9, 19, 13 at Addresses 20, 21, and 22, respectively.
Problem 3
- Try this program out:
0: LOAD [10]
STORE [11]
HALT
10: 55
11: 0
- Run it.
- Observe that when your program has finished running, it will have copied the number 55 from Location 10 to Location 11. Note that this is a copy of operation, and you end up with two locations containing 55.
- Modify your program so that it copies the 55 from Address 10 to the locations at Addresses 11, 12, and 13.
- Verify that your program works
- Put a different number at Address 10, and run your program again. Verify that this number is copied 3 times by your program to the three locations, at Addresses 11, 12, and 13.
Problem 4
- Try this program out:
LOAD [8] ;
ADD 1 ;
STORE [8] ;
HALT ;
8: 55 ;
- Run the program
- What does it do? Pay close attention to the number stored at Address 8 in memory. What is the result of the computation?
- Once you've figured it out, modify the program slightly so that Address 8 originally starts with the value 55 in it, and then ends with the value 57.
- Play with your program some more and verify that your program will add 2 to the variable at Address 8, whatever its original value.
- Once your programs works, modify it some more so that now it adds 2 to the contents of two memory locations, one at Address 20, and one at Address 21.
Problem 5
- Try this new program out:
0000: LOAD [10] ;
0002: ADD 1 ;
0004: STORE [10] ;
0006: JUMP 0 ;
0010: 55 ;
- Make sure Run the program. Make sure your speed is set to Slow. Study the various memory locations. In particular Address 10.
- Observe that your program never stops running. It loops forever. We call such a program an endless loop.
- Stop your program, and modify it so that the contents of Address 10 increases by 5 every step through the loop.
Mini Challenge of the Day |
Try this program out:
0000: LOAD [10]
0002: ADD [11]
0004: STORE [12]
0006: HALT
0010: 3
0011: 5
0012: 0
- Run it
- See what happens with Location 12.
- Do you see the relationship between the first 3 instructions? One could translate them in English as follows: "load the number stored in 10, add it to the number stored in 11, store the result in Location 12." In other words, the program computes Location12 = Location10 + Location11, if we give explicit names to the different memory locations.
- The challenge for you is to use this program as inspiration and write a new program that will compute the following: Location20 = Location20 x 2 + Location21. Make sure you initialize Locations 20 and 21 with numbers other than 0.
- Hints: You do not have to use the multiply operation!
THE END!
Solutions
P1
; Solution for Problem 1
LOAD 22 ; load 22 into the accumulator
STORE [10] ;
STORE [11] ;
STORE [12] ;
HALT ;
P2
; Solution for Problem 2
LOAD 9 ; load 22 into the accumulator
STORE [20] ;
LOAD 19 ;
STORE [21] ;
LOAD 13 ;
STORE [22] ;
HALT ;
P3
; Solution for Problem 3
0000: LOAD [10] ;
0002: STORE [11] ;
0004: STORE [12] ;
0006: STORE [13] ;
0008: HALT ;
0010: 55 ;
P4
- Part 1
LOAD [8] ;
ADD 2 ;
STORE [8] ;
HALT ;
8: 55 ;
- Part 2
0000: LOAD [20] ;
0002: ADD 2 ;
0004: STORE [20] ;
0006: LOAD [21] ;
0008: ADD 2 ;
0010: STORE [21] ;
0012: HALT ;
0020: 55 ;
0021: 13 ;
P5
; Solution for Problem 5
0000: LOAD [10] ;
0002: ADD 5 ;
0004: STORE [10] ;
0006: JUMP 0 ;
0010: 55 ;
Challenge
; Solution for Challenge
0000: LOAD [10] ;
0002: ADD [10] ;
0004: ADD [11] ;
0006: STORE [12] ;
0008: HALT ;
0009: HALT ;
0010: 3 ;
0011: 5 ;
0012: 0 ;